Python on the browser? Yes, it works!!
So far, if you wanted to do web development, there was only one choice - Javascript. But that changed last week. Well, at least in a small way. The hot news of the day was Anaconda announcing PyScript -- python on the browser with no install required. That's right, users just have to double click the html file and the python code will run on the browser, just like Javascript.
Wow, it actually works 🤯I just ran some python code in the browser **with no install required**. Just copy the code below, put it into a html file and open in the browser. That's it. https://t.co/OpyHqViGzY pic.twitter.com/oOrJulQCsP
— Playful Python (@playfulpython) May 1, 2022
Not convinced? Just copy the code below, paste it into an empty html file, and double click to open the file in a browser. You should get "Hello, python" displayed on your browser screen.
<html>
<head>
<link rel="stylesheet" href="https://pyscript.net/alpha/pyscript.css" />
<script defer src="https://pyscript.net/alpha/pyscript.js"></script>
</head>
<body>
<py-script>
print("Hello, python")
</py-script>
</body>
</html>
How??
Look at the code above once again. What is that py-script tag? This is not a regular HTML tag, but then how did the browser know to render it without any errors? The answer is web components.
What are web components?
Web components allow you to create your own components with your own tag name, which you can insert into your html pages wherever you want the component to be used. You might have seen a similar concept if you have ever coded in React, Vue or Svelte. For example the code below creates a custom component called World which can be inserted into the Hello component like a html tag
function Hello() {
return <div>Hello <World/></div>
}
function World() {
return <div>World</div>
}
The difference is that React components need React framework to run. They are not standardised components. While web components are standardised and you can use web components without requiring React or any other framework. This page on MDN gives more information on web components.
Web components are already supported by all the major browser. From MDN:
Web components are supported by default in Firefox (version 63), Chrome, Opera, and Edge (version 79). Safari supports a number of web component features, but less than the above browsers.
Moreover the mobile versions of the browsers also support it, so it will work on a phone as well.
Let us get back to the code. There is this script tag up on top
<script defer src="https://pyscript.net/alpha/pyscript.js"></script>
This tag loads PyScript. PyScript is the script which defines the <py-script> web component. We just need to include this script in the top and then we can use this new tag anywhere in our html file.
How does pyscript work?
Well, apart from creating the <py-script> web component, we also need to read the python code inside the tag, then parse it and run it. Normally we execute a python interpreter which does all that for us. We know that the browser comes built-in with a javascript interpreter, but how does it get access to a python interpreter?
Well, the <py-script> web component above contains the python interpreter and runs it on the browser.
What? It has the whole python interpreter? Answer is yes. This is not a variation or a stripped down version of python for the browser. This is the full, whole python interpreter.
But isn't the browser able to only run Javascript? Is python interpreter re-implemented in Javascript?
The answer is no, and no. To understand it further, we need to understand web assembly first.
What is Web Assembly
Web Assembly is a bytecode standard for a browser based virtual machine. That sounds a bit complex, so let us simplify it a bit.
Let us start with virtual machines, take the Java virtual machine as an example. All the Java code that we write gets compiled into "bytecode". These are special instructions that the Java Virtual Machine can execute. So you have source code -> byte code -> virtual machine executes the bytecode.
With such an architecture, you can have other languages which can also compile to the same bytecode. For example, Kotlin, Clojure, Scala, Groovy all these languages get compiled to JVM compatible bytecode and you can use the JVM to execute the code.
This is the basic idea of web assembly. It defines the bytecode standard and the virtual machine. Theoretically, you can take any code in any language and use a special compiler which can compile the code and give the output as the WASM bytecode. Once you have that, you can execute the code on the WASM virtual machine.
The good news is that all major browsers (and the mobile browser equivalents) already support WASM today. They have the virtual machine built in the browser, so any WASM bytecode can be run on the browser.
At this point there is a popular compiler for WASM called Emscripten. This compiler can output WASM bytecode, but it only takes C / C++ as input.
This is a problem if you want to write the code in python.
Getting python on to the browser
The python community found a creative fix for this problem. You see, CPython, which is the python interpreter that we get from python.org and we all use, is written in C. Which means that we can use Emscripten to compile the interpreter to WASM. And that is exactly what they did. The result is Pyodide, a version of Python that is compiled to WASM.
Once compiled, the python interpreter can be executed on the browser. And the python interpreter can execute the python code that is in the <py-script> tag.
So here is the sequence of steps
- Include a <script> which includes pyscript.js in your html file
- This js file defines a web component which creates the <py-script> tag
- When we use the <py-script> tag, load the contents inside the tag and give it to Pyodide. The browser will execute the python interpreter on the WASM virtual machine, which will run your code and show the output
- Output of your python code is displayed in the browser
The best part? The end user does not need to install anything (as long as they have internet connection to get pyscript.js. Even if there is no internet connection, you can download and zip up the javascript files along with your html file and the user can run it locally without Internet and without installing python.
Demo: Tic-Tac-Toe game
Below is a demo of the tic tac toe game written in python and running on the browser using pyscript. The whole game is a single html file -- there is no backend server processing to run the python code. You can save the html to your computer and run it from there if you want. You can also view source to see the python source code used to run the game.
Click this link to run the demo
Image of the demo game:
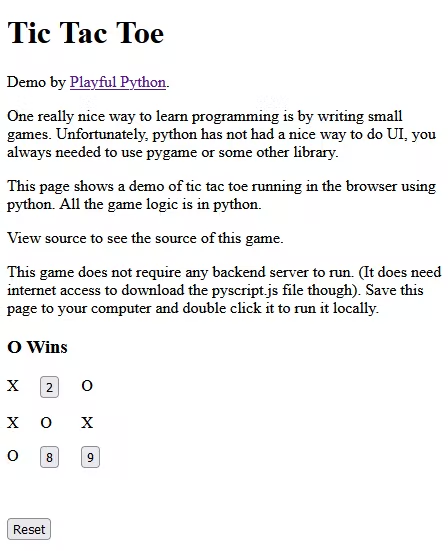
What all can we do with PyScript?
Pyodide includes the python interpreter and the entire standard library in the browser. Any code that uses these will run in the browser, including all new features like f-strings and asyncio. Pyodide has a mechanism through which other python libraries can also be installed and used on the browser. Pyodide and also packages standard data science packages (many of these are written in C as well). So you can use numpy, pandas, matplotlib and so on. This is a game changer for data science.
In the old world, to build a web visualisation you need to have a backend server (maybe written in python) but the interactive bits have to be coded in javascript, because that is all that the browser can understand. So data scientists have to learn two languages, which is a pain.
Also if you are distributing python code, then you have to ensure that the end user also has python downloaded and installed. Then they have to pip install all the libraries, hopefully they installed the correct versions. And then they can run your python script. This is very difficult when you want non-programmers to do all this.
Compare with someone just double clicks a html file and the JS code will run and show the visualisation in the browser itself, and the user can play around with it through the browser UI. If you want to use libraries, just put those js files in the same folder as the html and you can use it. Very simple.
PyScript includes data science libraries by default, this allows to build data visualisation tools directly in the browser. Yes, you can now use pandas and numpy and all those tools directly in the HTML!!!
And end user does not have to install anything. They don't need python installed on the system. No need to pip install pandas. Nothing. Just double click the html file and your app will run. Amazing!
PyScript also wraps certain popular JS libraries like the popular D3 visualisation library. This means you can actually call and use these JS libraries in your python code.
Limitations of pyscript
Pyscript is big - about 20 MB in size, so you can't use it where page loading speed is important or where you expect Internet connection to be very bad. Pyscript also takes a few seconds to start up after download. On the plus side, since everything is local, you can download pyscript previously and cache it aggressively to improve performance.
Future of web development?
Due to the above limitations, I don't see pyscript replacing any big JS framework like React or Vue anytime soon.
Still, web development is a vast area. Not everything is about complex frameworks, SSR, static pages, scaling etc. Even building a UI or tool in vanilla javascript, HTML and CSS is web development.
Until now, there was no option, web development meant learning javascript. But it will be difficult for data scientists to learn a new language just to build a front end visualisation to a pandas dataframe.
Of course PyScript is also a great option for teaching kids (or adults) to code. Python has always been a natural language for teaching coding, but the fact that you cannot build a web UI easily has been a major limitation because people like to see games and cool things happening on the screen. For these kinds of use cases also pyscript is a good option.
PyScript is still in very early alpha, so there are many rough edges. I expect these will get smoother as the months go by. And while this is still very, very early days, there is lots of potential in this project.